How to Send an SMS from AWS Lambda
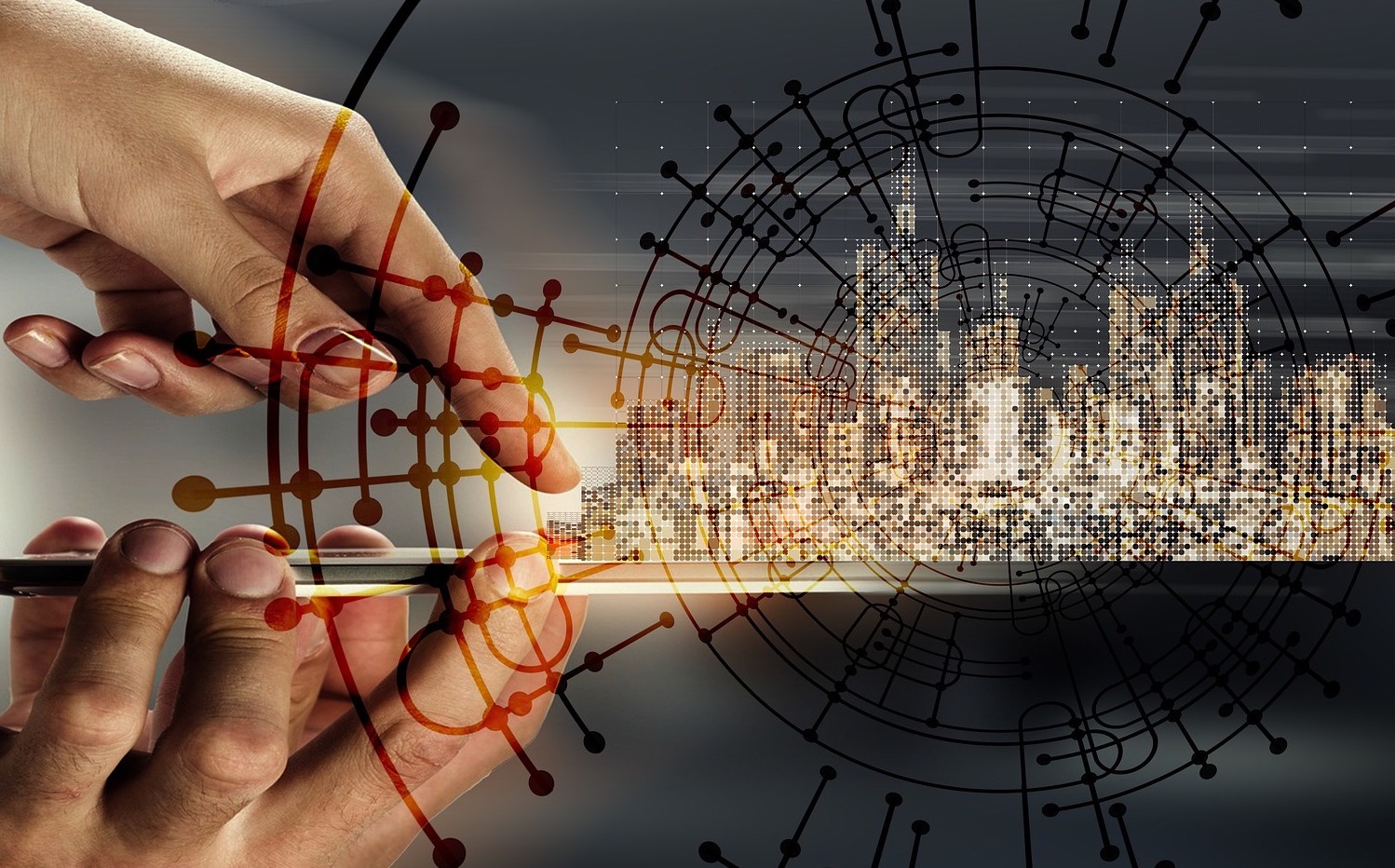
Amazon’s Simple Notification Service (SNS) can send a text message (SMS) to mobile phones almost anywhere in the world. But how can we do this programmatically? This article shows how simple it is to send an SMS from a Node.js Lambda function.
Let’s start by creating a Lambda function with the required permissions:
The SNS publish policy shown above, permits this function to perform the action “SNS:Publish” on resources “arn:aws:sns:*:*:*”. In our case, the resource will be a phone number, so it won’t match the resources expression. If you run the function now, it fails with an error:
{ "errorType": "AuthorizationError", "errorMessage": "User: arn:aws:sts::123456789012:assumed-role/sms-lambda-role/sms-lambda is not authorized to perform: SNS:Publish on resource: +123456789012" }
So open up this role in IAM & edit its policy to look like this:
{ "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Action": [ "sns:Publish" ], "Resource": "*" } ] }
Now, onto the code! The absolute bare minimum code required to get an SMS to your phone is shown below. It uses the JavaScript AWS SDK to publish to SNS. The publish params tell SNS that this is an SMS notification & not an email, etc.
var AWS = require('aws-sdk') var SNS = new AWS.SNS() var params = { PhoneNumber: '+123456789012', Message: 'SMS from Lambda!' } exports.handler = async (event) => { return new Promise(function(resolve, reject) { SNS.publish(params, function(err, data) { if (err) { console.log(err) reject(err) } else { console.log(data) resolve(data) } }) }) }
That’s it! That’s all it takes. Simple, isn’t it? You can learn more about SNS’s publish API here.
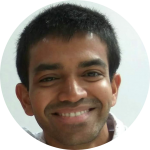
Harish KM is a Cloud Evangelist & a Full Stack Engineer at QloudX.
He is very passionate about cloud-native solutions & using the best tools for his projects. With 10+ cloud & IT certifications, he is an expert in a multitude of application languages & is up-to-date with all new offerings & services from cloud providers, especially AWS.